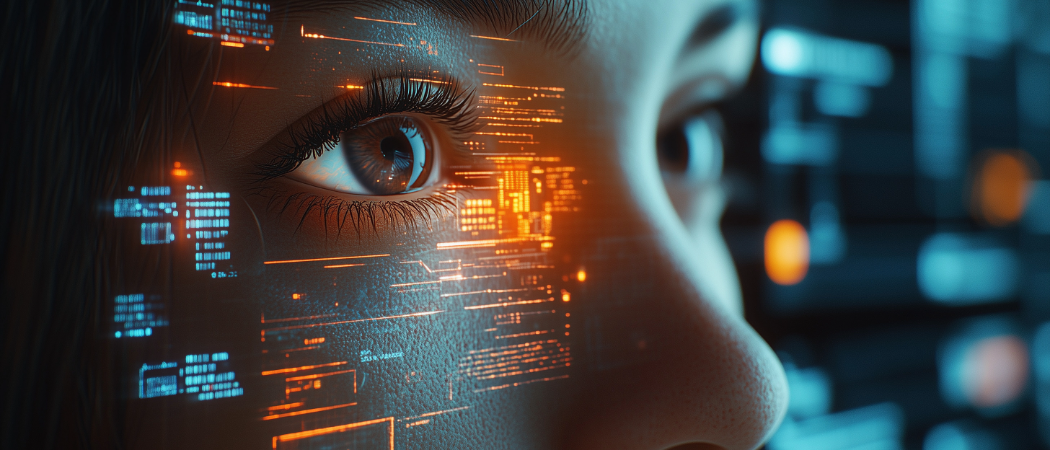
Laravel + Queues
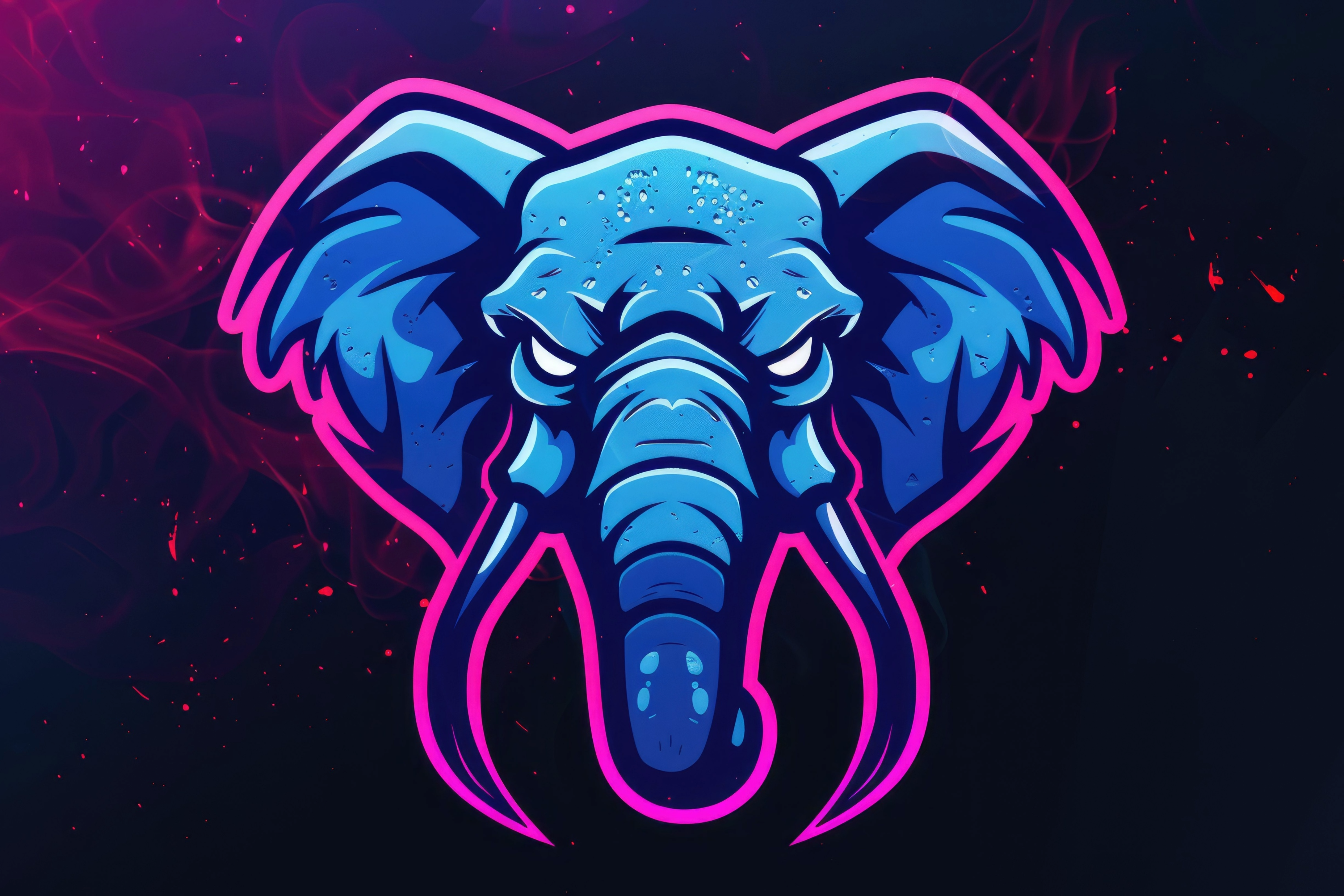
Don’t block your user while your server sends an email or calls an external API. In every Laravel project we build at Arudhra IT Techs, we rely on Laravel Queues to move heavy tasks out of the request cycle. From mail to PDF, third-party API syncs to order processing — this is how we run Laravel jobs in production.
Laravel + Queues: How We Handle Background Jobs at Scale (Mail, PDF, Webhooks & More)
In high-performance apps, background tasks aren’t optional — they’re essential. With Laravel Queues and Jobs, we push time-consuming work like email, PDF generation, webhook calls, and billing operations into the background — keeping the app fast, smooth, and scalable.
🚀 What Are Laravel Queues?
Laravel Queues let you defer time-heavy operations so they don’t block the user. This means:
- ✅ Better response times
- ✅ Safe retries and failure tracking
- ✅ Real-time processing for async tasks
Laravel supports queues via database
, Redis
, Amazon SQS
, Beanstalkd
, and more.
🔧 Common Use Cases We Handle with Queues
- 📧 Sending transactional or bulk emails
- 📄 Generating PDFs like invoices, reports, or receipts
- 🔗 Calling third-party APIs (payment, CRMs, SMS, etc.)
- 📥 Processing webhook events (like Stripe, Razorpay)
- 📊 Running exports or data analysis scripts
⚙️ Creating a Basic Laravel Job
Here’s how we define a simple email-sending job:
php artisan make:job SendInvoiceMail
// app/Jobs/SendInvoiceMail.php
public function handle()
{
Mail::to($this->user->email)->send(new InvoiceMailable($this->invoice));
}
Dispatch it like this:
SendInvoiceMail::dispatch($user, $invoice);
This job now runs in the background queue, not during the main request.
🔁 Setting Up Queues in Laravel
To start using queues:
- Set
QUEUE_CONNECTION=database
(or Redis) in.env
- Run:
php artisan queue:table
+php artisan migrate
- Start worker:
php artisan queue:work
📄 PDF Generation in Laravel Jobs
We often generate PDFs like invoices, receipts, or reports in the background using dompdf
or snappy
:
$pdf = PDF::loadView('pdf.invoice', ['invoice' => $invoice]);
Storage::put("invoices/inv-{$invoice->id}.pdf", $pdf->output());
This process runs in a job so the user doesn’t wait while it renders and saves.
🔗 Webhooks, API Calls, and External Services
API response times vary — so we defer them:
Http::post('https://api.thirdparty.com/push', [
'id' => $user->id,
'event' => 'order_completed'
]);
Using a queue job ensures we retry failed API calls and avoid crashes due to timeouts.
🛡️ Retry & Failure Handling
Laravel lets you control retries and failures:
public $tries = 5;
public $timeout = 20;
public function failed(Exception $exception)
{
Log::error('Job failed: ' . $exception->getMessage());
}
We also monitor failed jobs with php artisan queue:failed
and even send email alerts.
📊 Queue Monitoring in Production
- 🔁 Use Supervisor to run persistent queue workers
- 📈 Use Laravel Horizon for Redis queue UI + monitoring
- 🛎️ We set up Slack or email alerts for failed or stuck jobs
📦 Real Example: E-Commerce Order Processing
In one project, our Laravel backend:
- 🧾 Generated PDF receipts for every order
- 📬 Sent mail to customer + vendor
- 🔁 Synced stock count with 3rd party ERP via webhook
All of this was handled asynchronously using Laravel queue jobs — zero delay for the user.
Final Word: Laravel Queues Let You Build Like a Pro
Whether you're handling emails, APIs, or heavy tasks like PDF generation, Laravel Queues let you scale effortlessly without blocking your app. At Arudhra, we use them in every serious backend — and now, so can you.
Need help with queues, jobs, or scaling Laravel in production? We’ve got you 👇